Thoughts on the End of Wave.
Since my aliens don't "advance" to cover the screen, I'm concerned about the game-play during the time near the end of the wave of aliens. I don't want the situation where the payer can rest to the side with impunity, while a handful of aliens drop bombs impotently in the other corner.
Other games of this genre (ah... "alien shooting"), typically have a "Hurry Up" alien, like the small saucer in Asteroids or the "Baiter" in Defender, and even that stupid little bouncy smiley face[1] in Berzerk... Anyway the purpose of all these entities was to produce a fear in the player that they better get rid of the "easy" aliens quickly, before the much-more-deadly aliens came along.
So I'm toying with the idea of having the aliens do "bombing runs" when there's less than 10 remaining. Maybe there's a random 10% change of a bombing run per remaining alien. I wanted an interesting pattern for the flight-path, and have been playing with logarithmic spirals, just because they're pretty. So far I've hacked-up a demo in python which shows the movement points.
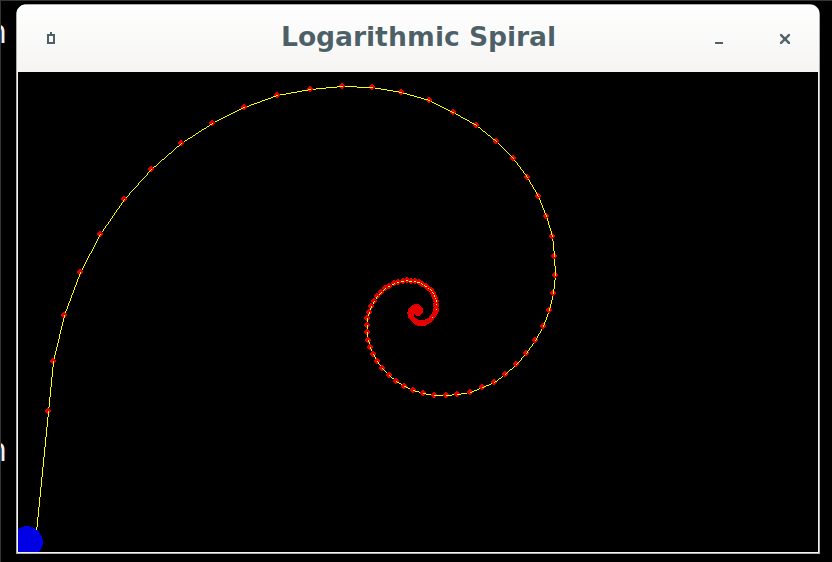
I'm hoping this will look good, but also provide a flight-path for the alien that makes it a little more difficult for the player. Also the player must react, as the alien is on a collision-course.
One problem with this path, is that it's not feasible to pre-generate points for every alien position per player position, so they'll have to be generated on the fly. The maths is reasonably hefty with calls to pow()
and trig' sin()
& cos()
functions. But I think so as long as it's not called too much, performance will be OK.
What do you think - is the spiral stupid? I really like its curves though.
--
[1] Honestly, who's idea was the smiley-face-of-doom??! It really doesn't fit the game world-theme at all. I'd wager it was hacked-in (or badly tweaked) at the last minute to ensure a better rate-of-return per hour with no concern for the gameplay. And it looks crap.
It's really fast, can walk through walls, and you can't shoot it. 