I have added a new version of the tutorial. This time, we will be dealing with light switches. Info down below
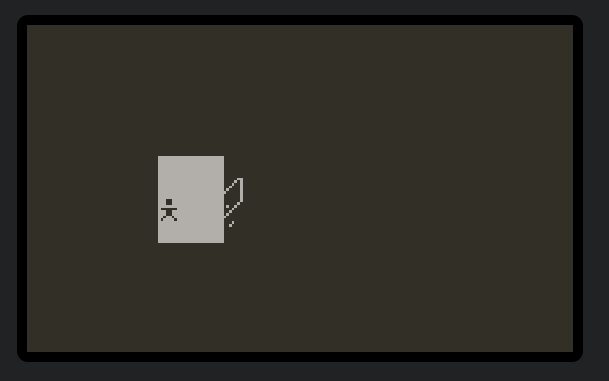
light_switch_demo.zip (3.1 KB)
How to add light swtiches: First, you are going to want to create 4 tiles, actually, just one, but we can duplicate it. You will want to make a light switch tile, duplicate it 3 more times and two of them should be flipped for the other room. For the light switch tiles, they should be called something like lightswitch_room1_on, lightswitch_room1_off, lightswitch_room2_on, and lightswitch_room2_off, just an example.
We are going to be removing the room code and instead adding that to the light switch tile code.
Also, we are going to want to create a new player tile called player_dark. You can duplicate your player tile, but invert the tile if you want. That is what I did.
lightswitch tile:
on enter do
playerType = "player"
tell "player" to
call "obeyType"
end
end
For the on switch tiles, you will want playerType to be "player" and for the off switch tiles, you will want the playerType to be "player_dark".
In the player code, we are going to want to add some more lines of code. When the player interacts enters a room with a on switch, the player will be the default player tile and when the player enters a room with a off switch, the player will be the player_dark tile.
on obeyType do
if playerType=="player" then
swap "player"
end
if playerType=="player_dark" then
swap "player_dark"
elseif playerType=="player_door" then
swap "player_door"
end
end
For the light switch tiles, you will want to use on interact do below your on enter do code.
Example code for the light switch:
sound "switch"
tell 9,9 to
swap "light_switch_off"
tell 6,6 to
swap "darkness"
tell 7,6 to
swap "darkness"
tell 8,6 to
swap "darkness"
tell 6,7 to
swap "darkness"
tell 7,7 to
swap "darkness"
tell 8,7 to
swap "darkness"
tell 6,8 to
swap "darkness"
tell 7,8 to
swap "darkness"
tell 8,8 to
swap "darkness"
tell 6,9 to
swap "darkness"
tell 7,9 to
swap "darkness"
tell 8,9 to
swap "darkness"
playerType = "player_dark"
tell "player" to
call "obeyType"
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
Woah there partner, what does all this do? First, you are telling the tile when you interact with it to play a sound effect. When interacting with a light switch, this should happen: sound effect plays -> light_switch_on is swapped with light_switch_off, light walkable tiles are swapped with dark walkable tiles -> player sprite gets swapped with player_dark
This is what I did for the light switch sound effect.
Full code for light_switch_on for the first room.
on enter do
playerType = "player"
tell "player" to
call "obeyType"
end
end
on interact do
sound "switch"
tell 9,9 to
swap "light_switch_off"
tell 6,6 to
swap "darkness"
tell 7,6 to
swap "darkness"
tell 8,6 to
swap "darkness"
tell 6,7 to
swap "darkness"
tell 7,7 to
swap "darkness"
tell 8,7 to
swap "darkness"
tell 6,8 to
swap "darkness"
tell 7,8 to
swap "darkness"
tell 8,8 to
swap "darkness"
tell 6,9 to
swap "darkness"
tell 7,9 to
swap "darkness"
tell 8,9 to
swap "darkness"
playerType = "player_dark"
tell "player" to
call "obeyType"
end
end
end
end
end
end
end
end
end
end
end
end
end
end
end
For the off switch for the first room, you will want to change all the lines that say "darkness" to "white" and for the on enter do section, change playerType = player
to playerType = player_dark
and for the playerType in the interact do section, change that from "player_dark" to "player".
For the first room you should have the light switches be called something like room1_lightswitch_on and the off switch tile to room1_lightswitch_off.
For the second room, have the two light switches be called something like room2_lightswitch_on and room2_lightswitch_off. The light switch tiles should be flipped depending if you are using a isometric perspective or something. Also, you will want to change the x,y coordinates for each tile you are swapping in the 2nd room, especially if the walkable tiles are in different spots. When you mouse over a tile in a room, you should see the x and y coordinates of that tile.
Hopefully this was a helpful guide. If you have any questions, suggestions, criticisms, or whatever, please let me know.