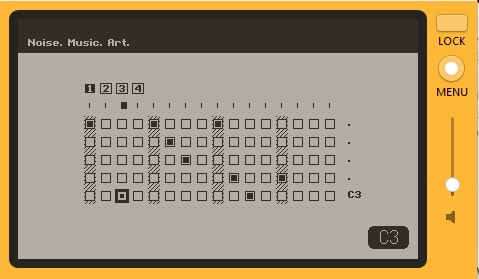
I'm working on a music step sequencer. 16 steps per sequence, and you can create multiple sequences.
It's really just 1 playdate.sound.sequence, but I loop it in a range (seq 1 is step 1-16, seq2 is step 17-32, seq3 is step 33-48 and so on), as just by looping the steps I avoid creating multiple synths/instruments/sequences. This works just fine, as the loop is set to repeat forever.
To make this more useful, the next step is a song mode, where you should be able to set what sequences you want to play (in what order, and whether they should be repeated). I don't know if this is standard, but you can find examples of this in multiple devices (teenage engineering Pocket Operators, Synthstrom deluge, m8 tracker, nanoloop). For example:
you have sequences 1,2,3,4
in song mode, you set it to play the sequences 1,1,2,1,3,4,2,1. This is where making a loop not continue to the end is very useful. As I'd do something like
local sequences = {1,1,2,1,3,4,2,1}
local currentSeq=0
function playThisThing()
currentSeq = currentSeq+1
local currentStep = (sequences[currentSeq]-1)*16
sequence:setLoops(currentStep, curerntStep+15, 1)
sequence:play(playThisThing)
end
(I didn't test this code, but I think it shows the idea that rather than simply play a sequence, you can control when and how long to play a certain part of it).
Something like this would also allow to create effects (shortening loops for stutter, move randomly in the sequence for granular)
The easy alternative is to create a new playdate.sound.sequence when in song mode, where I'd copy the notes I need from the original sequence, in the order I need them. I feel like this would be a bit limiting, because the fun would be in continue modifying sequences, reordering, deleting live.
I haven't tested this yet, but something I know is that sometimes when you add a note in a sequence that is playing, it plays that note immediately (like, if the sequence is playing and is on step 3, and you add a note on step 5, it plays that new note, while it continues to step 4).
The other alternative is to monitor the sequence current step in a loop, but this is very innacurate, as the playdate timer doesn't run at the same rate as the sound timer, so when I find that a sequence is on step 16, it might be already a few milliseconds into that step, so moving it to step 32 messes the tempo (also, when developing, I noticed that moving to a step when the sequence is playing stops the sequence, not sure if that's still the case).
setPlayRange() would definitely solve what I'm trying to do.
sorry for the long reply.