Hi there, I am attempting to create an animated mugshot above the typewriter-effect textbox where the character blinks and moves his mouth as text is being typed, then closes his mouth and blinks occasionally as a result of being idle. My first problem is how to make the image table used to represent the mugshot and animate it as the text is being displayed.
This is what it should look like on screen.
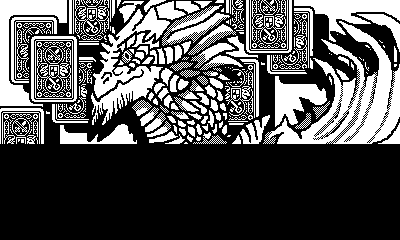
Also is there a possibility of adding new lines of text upon pressing the A button?
Thank you in advance. Also I'm using @neven 's typewriter.lua script, credit goes to him for providing it to fellow developers.
1 Like
There are many ways to achieve this.
I would:
- make the dragon character a sprite
- give it a
state
variable to store idle/talking
- give it variables for
image_body
, image_eyes
, image_mouth
, where each of these is a sprite (if static) or AnimatedSprite
- define
function update
for each of these image components that checks state
and sets the image/animation appropriately
Typewriter.lua is a demonstration, not an all-purpose library. If you want to display different text each time you press
, you’ll need to replace this:
function playdate.AButtonUp()
textbox.text = "Hello from the text sprite!"
textbox:add()
end
How you handle it will depend on how extensive/deep your dialogue options run, but for a simple example, you could do something like (untested):
local dialogue_position = 1
local dialogue = {“Hello”, “I am dragon”, “My breath is unpleasant”}
Then in update
if playdate.buttonJustPressed(‘a’) then
textbox:remove()
if dialogue_position == #dialogue then
— all dialogue has been shown, so add code to escape/do something else
else
textbox.text = dialogue[dialogue_position]
textbox.currentChar = 1
textbox:add()
dialogue_position += 1
end
end
Better yet - store the dialogue variables in the dragon sprite, then put the dialogue drawing logic in the dragon sprite’s update function.